Understanding the JavaScript Engine: Call Stack, Event Loop, Micro-tasks, and Macro-tasks
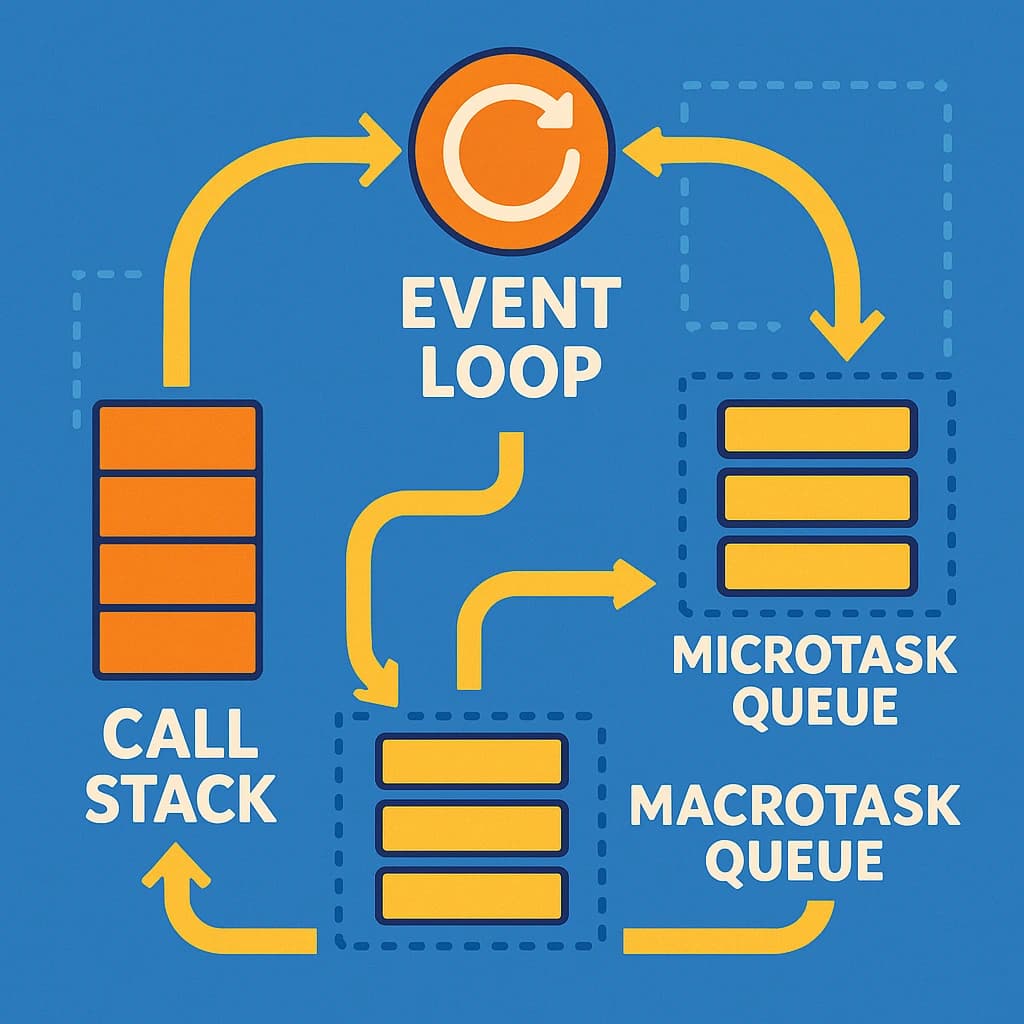
[IMAGE_PLACEHOLDER]
JavaScript is known for its non-blocking, single-threaded behavior, powered by the JavaScript engine and its event loop. Understanding how these components work is crucial for writing performant and responsive applications.
In this post, we’ll delve into the call stack, event loop, macro-tasks, micro-tasks, and the JavaScript engine that makes it all possible.
The JavaScript Engine
A JavaScript engine, such as V8 (used in Chrome, Node.js, and React Native) or Hermes (used by React Native for Android), is the core component that executes JavaScript code.
Key Responsibilities of a JavaScript Engine
- Parsing JavaScript Code:
- Converts the source code into an Abstract Syntax Tree (AST).
- Compilation/Interpretation:
- Modern engines use Just-In-Time (JIT) compilation or Ahead-of-Time (AOT) compilation for efficient execution.
- Execution:
- Executes the machine code generated during compilation.
- Memory Management:
- Handles garbage collection, memory allocation, and optimization.
The Call Stack
The call stack is a critical part of the JavaScript engine. It is a Last In, First Out (LIFO) data structure that keeps track of all function calls and ensures synchronous code is executed in order.
How the Call Stack Works
- Pushing Functions:
- When a function is called, it is added to the top of the stack.
- Popping Functions:
- When a function finishes execution, it is removed from the stack.
- Blocking the Stack:
- Long-running synchronous operations block the stack, preventing other code from executing.
Call Stack Example
function greet() { console.log('Hello!') } function sayGoodbye() { console.log('Goodbye!') greet() } sayGoodbye()
Execution:
sayGoodbye()
is pushed onto the stack.- Inside
sayGoodbye()
,console.log("Goodbye!")
runs. greet()
is called and pushed onto the stack.- Inside
greet()
,console.log("Hello!")
runs and the function is popped off. - Finally,
sayGoodbye()
is popped off.
Output:
Goodbye!
Hello!
The Event Loop
The event loop coordinates asynchronous and synchronous code execution, ensuring that non-blocking operations like timers and network requests don’t freeze the main thread.
How It Works
- Synchronous Code:
- Executes first on the call stack.
- Micro-tasks:
- Processed immediately after synchronous code finishes.
- Macro-tasks:
- Executed after the micro-task queue is emptied.
This prioritization allows high-priority tasks like resolved Promises to execute before lower-priority tasks like setTimeout
.
Micro-tasks vs. Macro-tasks
Micro-tasks
- High Priority:
- Executed immediately after the call stack clears.
- Examples:
Promise.then()
queueMicrotask
MutationObserver
Macro-tasks
- Lower Priority:
- Executed after all micro-tasks in the same cycle are completed.
- Examples:
setTimeout
setInterval
- File I/O and network callbacks
Execution Order of Tasks
Let’s analyze how the event loop prioritizes tasks:
console.log('Start') setTimeout(() => console.log('Macro-task: setTimeout'), 0) Promise.resolve().then(() => console.log('Micro-task: Promise')) console.log('End')
Step-by-Step Execution
- Synchronous Code:
console.log('Start')
runs first.
- Scheduling Tasks:
setTimeout
schedules a macro-task.Promise.resolve()
schedules a micro-task.
- Finishing Synchronous Code:
console.log('End')
runs.
- Processing Micro-tasks:
- The micro-task queue processes the Promise callback.
- Processing Macro-tasks:
- The macro-task queue processes the
setTimeout
callback.
- The macro-task queue processes the
Output:
Start
End
Micro-task: Promise
Macro-task: setTimeout
Why Understanding These Concepts Matters
Call Stack
- Knowing how the stack works helps avoid blocking operations that can freeze an application.
Event Loop
- The event loop’s scheduling of micro-tasks and macro-tasks directly impacts application performance.
JavaScript Engine
- Understanding how engines parse, compile, and execute code helps debug performance bottlenecks and optimize critical code paths.
Summary
The JavaScript engine, call stack, event loop, micro-tasks, and macro-tasks are the foundation of how JavaScript executes code. Mastering these concepts allows you to write efficient, responsive, and predictable applications, whether in the browser, Node.js, or frameworks like React Native.
By understanding these key mechanics, you can confidently debug complex asynchronous code and build applications that perform seamlessly under heavy workloads.